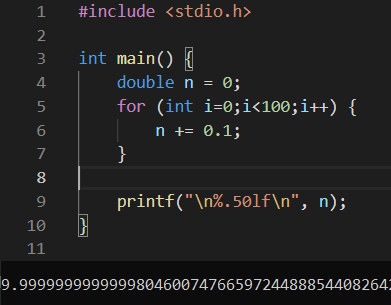
Accurate Decimal/Big Number Addition using C
Decimal calculations can be inaccurate on most computers. When adding float or double type numbers, there can be some unwanted digits in the results. (example on the left)
Our team designed different algorithms to complete this task, with each of us attempting to solve this challenge with different algorithms.
We first tried to solved it by converting the decimal to an integer. Then simply add them together. The problem of converting decimals to integers is that if there are any big numbers, then they would be too large to store in integer or long type variables.
One way of solving it was adding each digit according to the place value without aligning the decimal points. However, we found that aligning the decimal points, then add the numbers together is faster and easier.
So we first converted the digits to a char array. Next, out team aligned the decimal point by moving the decimal point to the center of the array.
// loop through two string
Last, we add them starting from the last digit of both number. Thus, we can accurately add decimals or big numbers without any error.
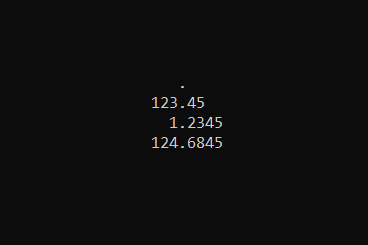