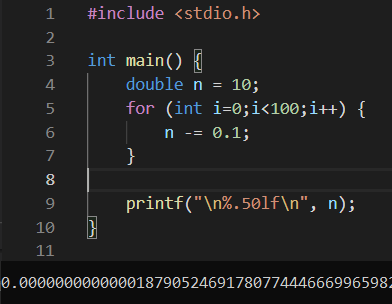
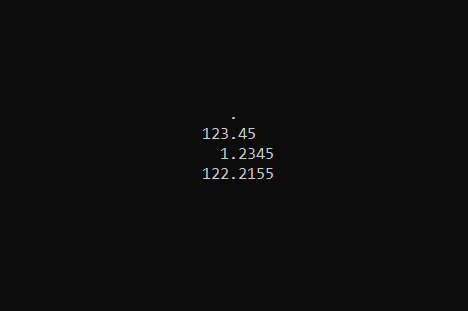
Accurate Decimal/Big Number Subtraction using C
After solving decimal/big number addition, our team moved on to try to accurately subtract decimals without any errors.
Our first thoughts were to simply change the addition sign to a subtraction sign. However, this would create a wrong result if the correct result is a negative number.
One way of solving this was by adding a 1 in front of the first number when the second number is bigger. Then, subtract that number from 100. Lastly, add a negative sign in front of the result.
For example, 12 – 34. First add a 1 in front, ‘1’12 – 34 = 78. Then, subtract 78 from 100, 100 – 78 = 22. Therefore, 12 – 34 = -22.
Another way of subtracting a bigger number from a smaller number is to find the larger number; then, subtract the smaller number from the bigger number.
Below is an example code of how to find the larger number